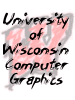 CS559
Fall 2005
News
Calendar
Assignments
Lectures/Notes
Tutorials
Sample Code
Project 1
Project 2
Project 3
Basic Info
Policies
C++ hints
|
Update 11/12/2005:
A Project 2 Grading clarification For different features that have multiple
parts, sometimes the parts do add up.
- Having both rail ties and parallel rails is worth more than having
either one, but probably not as much as having both (if it were really
amazing you could get all 17 points, but it would have to be amazing).
Just drawing a line for the rails and drawing lines for the rail ties
is a simple way to do "fancy tracks" (better is to draw polygons to
give the them some thickness).
- The other "multiple parts of one things" vary. simple physics would
be 5 points for momentum, and 5 more points for having the "ramp up".
- for multiple cars, 10 points is for having multiple cars on the track
at the same time with the correct spacing. 5 additional points (for
a total of 15) if the cars are different designs.
You should also be aware that the point values are for doing things correctly.
You will get some subset of the points for doing simpler variants.
Project 2: Trains and Roller Coasters!
Due Tuesday, November 15th, 9:30am.
(note: the due date was moved back from November 8th)
Late assignments accepted according to the course
late policy.
Overview
In this project, you will create a train that will ride around on a track.
When the track leaves the ground, the train becomes more like a roller
coaster.
The two main purposes of this project are to give you experience in working
with curves (e.g. the train and roller coaster tracks) and to give you
experience with writing interactive 3D programs.
In previous years, this assignment has emphasized the construction of
the user interface to specify the train tracks.
The core of the assignment requires you to create a 3D world, and to
allow the user to place a train (or roller coaster) track in the world.
This means that the user needs to be able to see and manipulate a set
of control points that define the curve that is the track, and that you
can draw the track and animate the train moving along the track. The first
part (making a world, manipulating points) was a Programming Assignment,
and we gave you sample code.
You are to write this program doing the drawing with the OpenGL graphics
library under Windows. You may use whatever user interface tools you wish
(we recommend FlTk, but GLUT would be an acceptible alternative). See
the GL Survival kit for thoughts on this.
There is a lot of available sample code, but you do not have to use it.
There are two example solutions to this project in P:/course/cs559-gleicher/public/bin,
both from 1999. One is a version that I wrote (called mikes-Train) and
another was written by a really good student (robs-train). I recommend
that you try them out to get an idea as to what you'll be doing.

Mike's sample train, circa 1999 |
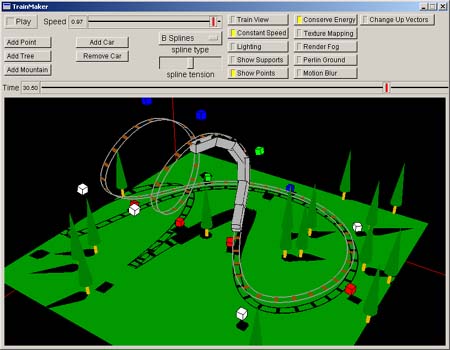
Rob Iverson's A+ assignment from 1999 |
While the assignment was a little bit different in 1999, the basic idea
was the same. For a totally crazy solution to this assignment, check out
RocketCoaster.
It was what happened when I let two students work as a team. And they
were really good students.
The Parts of The Assignment / Grading
The basic part of this assignment is to provide a train going around
the track. You must:
- Provide a user interface to look around the world.
- Provide a user interface that allows control points to be added, removed,
or repositioned. Note: even if you do a very advanced interface, you
should display the control points and allow for them to be edited manually.
- Have a track that is (at least) C1. Your program should draw the track.
- Have a train that goes around the track (with a play button to start/stop
it). The train should always be on the track. Your train need not be
fancy, but it should be obvious which end is the front.
- Have the train oriented correctly on the track. The train should always
face forward if the track is flat, and mostly face forward on a 3D track
(some cases, such as vertical tracks or loops can be hard, a basic assignment
doesn't have to get those right).
- Allow the user to "ride" the train (look out from the front
of the train).
- Have some scenery in the world besides the groundplane.
- Use lighting.
- Your program is properly documented, is turned in correctly, and has
sufficient instructions on how to use it in the readme file.
If you're curious, my sample solution (mikes-train.exe) does not do all
of these. (the train has front, and the only scenery is the point light
source - also, there's a wierd bug where the train stretches out when
it goes down a hill).
If your assignment does all of these basic parts, you will get at least
a C. If your assignment doesn't do these basic parts, we will not look
at its advanced features.
Advanced features (discussed below) get you "advanced points"
which determine your grade above a C. But remember: if your basic features
don't work, you can't get any advanced points.
0-25 pts = BC
26-50 pts = B
51-75 pts = AB
76-100 pts = A
more than 100 pts = Extra Credit (although, your grade is an A)
If your code or documentation is bad, we may assess up to a 20 point
penalty. (This penalty cannot pull you below zero).
Advanced Features
You may pick as many advanced features as you want to implement. If you're
happy with a C, you don't need to implement any. Otherwise, pick as many
as you want to get enough points.
We will only check the features that you say that you have implemented
correctly. Partial credit will be given for advanced features, but negative
credit may be given for really incorrect features. (so, its better to
not say you implemented a feature than to show us something that is totally
wrong).
- Arc-Length Parameterization (15pts)
- Having your train move at a constant velocity (rather than moving
at a constant change of parameter value) makes things better. Implementing
this is an important step towards many other advanced features.
You should allow arc-length parameterization to be switched on and off
to emphasize the difference.
- Give a tension control for the splines (5 pts)
- This is so simple, but it will give you some intuitions for what the
tension parameter does. For cardinal splines, you could have a different
tension value for each control point.
- C2 Curves (10 pts)
- Having C2 Curves are better than C1 curves. (the 2nd order discontinuities
would make the motion unrealistic). With C2 curves, your train may not
go through the control points, but only approximate them.
- Direct Manipulation of C2 Curves (10 pts)
- If you implement C2 Curves that are approximating, you might try to
implement some scheme where the user manipulates the track and the control
points are adjusted automatically. One relatively easy method for doing
this is the paper:
Barry Fowler, Richard Bartels. "Constraint-Based Curve Manipulation,"
IEEE Computer Graphics and Applications, vol. 13, no. 5, pp.
43-49, September/October, 1993. If you're on the UW campus, you can
get the article here.
Note: to get full credit for this feature, you need to provide for total
control over the curve - just translating a control point when the user
tries to move the curve doesn't apply.
- Draw nicer looking tracks (10 pts for parallel rails, 7 pts for rail
ties)
- Drawing rail ties (uniformly spaced lines perpindicular to the tracks)
and parallel tracks is a little bit tricky. The rail ties require good
arc-length parameterization (to get uniform spacing). For parallel rails,
simply offsettign the control points doesn't work.
- Correct Orientation in 3D (5pts for looping correctly, 10 pts for
"up" controls)
- The simple schemes for orienting the train break down in 3D - in particular,
when there are loops. Make it so that your train consistently moves
along the track (so its under the track at the top of aloop).
One good way to provide for proper orientations is to allow the user
to control which direction is "up" at points along the curve.
This allows you to do things like corkscrew roller coasters.
- Save and Load Tracks (7 pts)
- Being able to write out track designs and read them back in makes
it easier to show off what your program can do.
- Have Real Train Wheels (8 pts)
- Real trains have wheels at the front and back that are both on the
track and that swivel relative to the train itself. If you make real
train wheels, you'll need arc-length parameterization to keep the front
and rear wheels the right distances apart (make sure to draw them so
we can see them swiveling when the train goes around a tight turn).
- Have Multiple Cars on Your Train (10 pts, 5pts for different designs)
- Having multiple cars on your train (that stay connected) is tricky
because you need to keep them the correct distance apart. You also need
to make sure that the ends of the cars are on the tracks (even if the
middles aren't) so the cars connect.
If you're really into trains, you could have different kinds of cars.
In particular, you could have an engine and a caboose.
- Have People On Your Roller Coaster (5pts)
- Little people who put their hands up as they accelerate down the hill
are a cool addition. (I don't know why putting your hands up makes roller
coasters more fun, but it does).
- Implement simple physics (5pts for momentum, 5 more for roller coaster
physics)
- Roller coasters do not go at constant velocities - they speed up and
slow down. Simulating this (in a simple way) isn't that difficult, and
would make for a good advanced feature. Remember that Kinetic Energy
- Potential Energy should remain constant (or decrease based on friction).
This lets you compure what the velocity should be based on how high
the roller coaster is.
Even Better is to have "Roller Coaster Physics" - the roller
coaster is pulled up the first hill at a constant velocity, and "dropped
" where it goes around the track in "free fall." You
could even have it stop at the platform to pick up people
.
- Have a "sketch-based" interface (10-20 points, depending
on
- Make an interface where the user draws a curve on the ground, and
figure out how to fit a spline to it.
If you're really interested in this, there are methods for sketching
3D curves (such as sketching 2D at a time). Ask us.
- Something so cool we can't predict it
- Yes, you might think of something to do that we didn't mention here.
If its really cool, we might give you points for it. We'd like
you to focus on trying to do more with the curves aspect of this assignment
(rather than making arbitrary eye-candy). If you want to do something
and you want to make sure it will be worth points, send the instructor
email.
Documentation
Your documentation must have 4 parts:
- User Documentation
- In a file called user.txt (or user.htm, if you want to make it a web
page so you can include pictures - just make sure all the links are
local and the pictures are included) you must explain how to use the
program.
- Technical Documentation
- In a file called technical.txt (or technical.htm, if you want to make
it a web page), you must list all of the advanced features that you
have implemented, as well as a brief description of how you implemented
in. Tell us the technique you used. Sometimes, this is simple ("C2
Curves: I implemted Uniform, Periodic, Cubic B-Splines"), or you
might have some explaining to do (say, if you make a sketch-based interface).
Your list should only include the features that work (the ones you want
us to grade). When we do the testing of your program, we will only try
out the features listed.
- Readme.txt
- You should have the normal readme file, explaining where everything
is.
-
Some Hints
In case it isn't obvious, you will probably use Cardinal Cubic splines
(like Catmull-Rom splines). Cubic Bezier's are an option (just be sure
to give an interface that keeps things C1. For the C2 curves, Cubic B-Splines
are probably your best bet.
You should make a train that can move along the track. The train needs
to point in the correct direction. It is acceptable if the center of the
train is on the track and pointing in the diretion of the tangent to the
track. Technically, the front and back wheels of the train should be on
the track (and they swivel with respect to the train). If you implement
this level of detail, please say so in your documentation. It will look
cool.
In order to correctly orient the train, you must define
a coordinate system whose orientation moves along with the curve. The
tangent to the curve only provides one direction. You must somehow come
up with the other two directions to provide an entire coordinate frame.
For a flat track, this isn't too difficult. (you know which way is up).
However, when you have a roller coaster, things become more complicated.
In fact, the sample solution is wrong in that it will break if the train
does a loop.
The sample solution defines the coordinate frame as follows:
(note: you might want to play with the sample solution to understand the
effects of this)
- The tangent vector is used to define the forward (positive Z) direction.
- The "right" axis (positive X) is defined by the cross product of
the world up vector (Y axis) and the forward vector.
- The local "up" axis is defined by the cross product of the first
two.
Doing arc-length parameterizations analytically is difficult for cubics.
A better approach is to do them numerically. A simple way to do it: create
a table that maps parameter values to arc-lengths. Then, to compute a
parameter value given an arc length, you can look up in the table and
interpolate.
|